
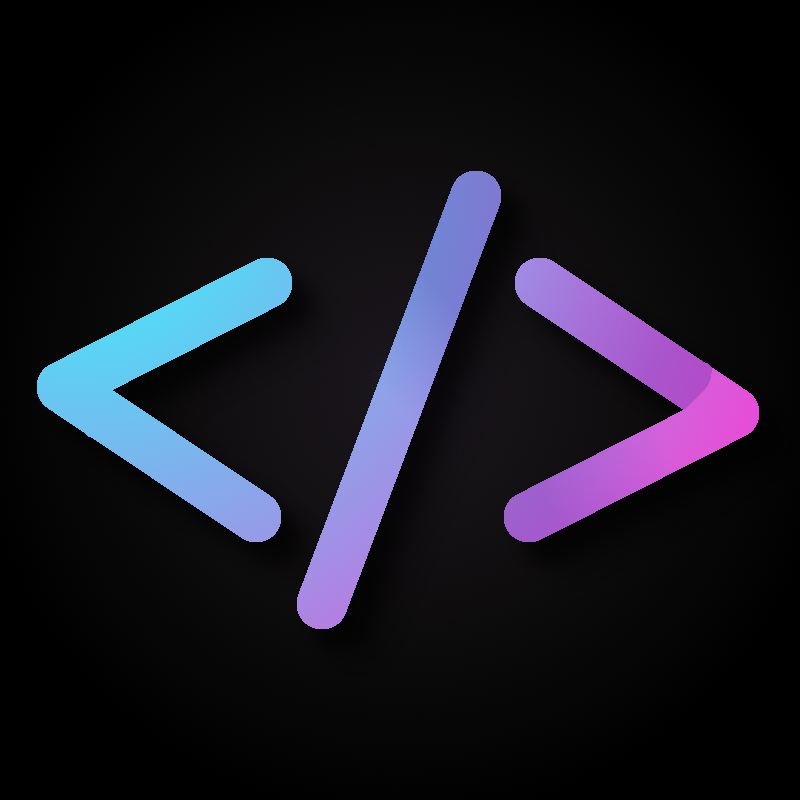
Speaking as someone with a MTF close friend and NB spouse, but the term used in the article is the term everyone around me used when I was growing up. That term may be obsolete now, and if so, the author simply needs to be informed. There’s no need to assume they meant harm by it.
If they knowingly used a term that may offend, then that’s of course a separate issue.
Ignoring the rest, just some thoughts about the list of proposed features:
Automatic implicit cloning would be useful for high level developers, but not ideal at all for low level or performance-sensitive code. It’s not the case that anyone using a shared pointer wants to clone it all the time. The high level usecase doesn’t justify the cost assumed by the low level users.
Instead, being able to wrap those types with some kind of custom “clone automatically” type feels like a middle ground. It could be a trait like mentioned, or a special type in the standard library. Suppose we call it
Autoclone[T]
or something (using brackets because Lemmy nonsense).Autoclone[Rc[T]]
could function like the article mentioned.Having “private” and non-“private” methods function differently feels like confusing behavior that should be avoided if possible. Also, “private” I assume refers to
pub(self)
methods (the default if unspecified), which is “module-level” methods (so accessible within the module it’s defined in). Anyway, there are years of discussion around this so I’ll just defer to that as to why it’s not in yet.I agree with the urge to make it happen though. Some method of doing partial borrows for methods would be nice.
This is what prompted me to even comment. What “every language” does for complex constructors is different per language. C#, for example, supports both named and optional parameters, but construction usually uses an object initializer:
This is similar to Rust’s initializers:
Where it gets tricky is around required parameters. Optional ones don’t really matter since you can use the syntax above if you want, or chain methods like with the builder style.
As for the overhead of writing builders, there’s already libraries that let you slap
#[derive(Builder)]
on types and get a builder type automatically.As for optional parameters, how those are implemented differs between languages. In C#, default values must be constant values. In Python, default values are basically “global” values and this nonsense is possible:
Anyway, all this is to say that the value of optional parameters isn’t obvious.
Named parameters is more of a personal choice thing, but falls apart when your parameter has no name and is actually a pattern:
Also, traits often use names prefixed with underscores in their default fn impls to indicate a parameter an implementer has access to, but the trait doesn’t use by default. Do you use that name, or the name the implementer defined? I assume the former since you don’t always know the concrete type.
We have that, it’s called the
try
operator.Okay I know it’s different, and I know everyone’s use case is different, but I’ve been coding long enough to know that enabling easy unwraps means people will use it everywhere despite proper error handling being pretty dang important in a production environment.
Thinking of my coworkers alone, if we were to start writing Rust, they’d use that operator everywhere because that’s what they’re familiar with coming from other languages. Then comes the inevitable “how do I add a try-catch block?” caused by later needing to handle an error.
Anyway, I prefer the extra syntax since it guides devs away from using that method over propagating the error upwards. For the most part, you can just use
anyhow::Result
and get most error types converted automatically.Yes please.
Yes please.
I’d want input from runtime devs on this, but if possible, yes please.
???
How is the compiler going to know how to compile the code if it doesn’t know the types? This isn’t Python. The compiler needs to know things like how much memory to allocate, and there’s a ton of potential unsound behavior that can occur from treating one type as another, even if they’re the same size.
Anyway I’ll save the rest for later since I’m out of time.